Hello,
I'm having problem with long strings in my win32 project. For some reason it just stops reading it after a string being x length long. Here is the code:
This is the current result:
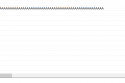
Any help appreciated.
I'm having problem with long strings in my win32 project. For some reason it just stops reading it after a string being x length long. Here is the code:
Code:
[COLOR=#000000][FONT=Inconsolata]HWND hListViewLog;[/FONT][/COLOR]LVCOLUMN lvLogList;
LV_ITEM lvLogItem = { [COLOR=#0000DD][B]0[/B][/COLOR] };
#[COLOR=#008800][B]define[/B][/COLOR] [COLOR=#880000]ListViewLog[/COLOR] [COLOR=#0000DD][B]400[/B][/COLOR]
[COLOR=#008800][B]void[/B][/COLOR] [COLOR=#880000]setListViewList[/COLOR](HWND hListView, LVCOLUMN lvList, [COLOR=#008800][B]int[/B][/COLOR] iSize, [COLOR=#008800][B]int[/B][/COLOR] iItem, wchar_t *wcName) {
lvList[COLOR=#003377].mask[/COLOR] = LVCF_FMT | LVCF_WIDTH | LVCF_TEXT | LVCF_SUBITEM;
lvList[COLOR=#003377].fmt[/COLOR] = LVCFMT_LEFT;
lvList[COLOR=#003377].iSubItem[/COLOR] = iItem;
lvList[COLOR=#003377].cx[/COLOR] = iSize;
lvList[COLOR=#003377].pszText[/COLOR] = wcName;
[COLOR=#CC0000][B]ListView_InsertColumn[/B][/COLOR](hListView, iItem, &lvList);
}
hListViewLog = CreateWindowEx([COLOR=#003388][B]NULL[/B][/COLOR], WC_LISTVIEW, [COLOR=#003388][B]NULL[/B][/COLOR], WS_CHILD | WS_VISIBLE | WS_BORDER | LVS_REPORT, [COLOR=#0000DD][B]25[/B][/COLOR], [COLOR=#0000DD][B]25[/B][/COLOR], [COLOR=#0000DD][B]745[/B][/COLOR], [COLOR=#0000DD][B]300[/B][/COLOR], hWnd, (HMENU)ListViewLog, ((LPCREATESTRUCT)lParam)->hInstance, [COLOR=#003388][B]NULL[/B][/COLOR]);
[COLOR=#880000]SendMessage[/COLOR](hListViewLog, LVM_SETEXTENDEDLISTVIEWSTYLE, LVS_EX_FULLROWSELECT | LVS_EX_GRIDLINES, LVS_EX_FULLROWSELECT | LVS_EX_GRIDLINES);
[COLOR=#880000]setListViewList[/COLOR](hListViewLog, lvLogList, [COLOR=#0000DD][B]10000[/B][/COLOR], [COLOR=#0000DD][B]3[/B][/COLOR], L[COLOR=#DD2200]"Log"[/COLOR]);
lvLogItem.iItem;
[COLOR=#880000]ListView_InsertItem[/COLOR](hListViewLog, &lvLogItem); [COLOR=#880000][FONT=Inconsolata]ListView_SetItemText[/FONT][/COLOR][COLOR=#000000][FONT=Inconsolata](hListViewLog, lvLogItem.iItem, [COLOR=#0000DD][B]3[/B][/COLOR], L[COLOR=#DD2200]"AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAABBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBCCCCCCCCCCCCCCCCCCCCCCCCDEFG"[/COLOR])[/FONT][/COLOR][COLOR=#000000][FONT=Inconsolata];[/FONT][/COLOR]
This is the current result:
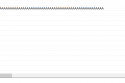
Any help appreciated.