Hello fellow programmers!
I will note that this is part of an assignment, so I don't want you to give me the answer outright, I just need some hints to help me find the solution. All advice is welcome!
So, as part of the project, I must create a program that can save and store ingredients for different recipes in VB.NET. For the data storage, I would like to use XML files because I feel that it is an efficient way to do so, but please suggest an alternative if you feel one is suitable.
So, the XML file I am testing with is as follows:
You can see that I have multiple recipes and the ingredients as child tags.
My form currently looks like this:
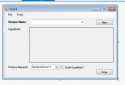
The program consists of a dropdown box for the user to select recipes, a DatGridView control to view/edit the ingredients, an up/down selection box to allow them to enter portion amounts (along with a checkbox that when checked, will scale up the quantities in the ingredients according to the new amount of people selected).
Currently, I have a way to view all the recipes in combobox, and can view either all the ingredients in the XML file in the DataGridView, or all the recipe names/portions in the DataGridView.
I need a way to filter the selected ingredients to only show the ones related to the one selected in the drop down.
My current code is below. Please note, this is no where near finished, but just used so I can get an idea of the techniques I need to use before I create the final program in school.
My changing the index in this line from 0 to 1:
I can either view all the recipe names, or all the ingredients for all the recipes.
I've hit a brick wall with this, and need all the help I can get.
Many, many thanks!
Stephen
I will note that this is part of an assignment, so I don't want you to give me the answer outright, I just need some hints to help me find the solution. All advice is welcome!
So, as part of the project, I must create a program that can save and store ingredients for different recipes in VB.NET. For the data storage, I would like to use XML files because I feel that it is an efficient way to do so, but please suggest an alternative if you feel one is suitable.
So, the XML file I am testing with is as follows:
Code:
<?xml version="1.0" standalone="yes"?>
<UserRecipes xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<recipe name="Chocolate" portions="5">
<ingredient quantity="500" unit="g">Chocolate Bar</ingredient>
<ingredient quantity="300" unit="g">Chocolate Buttons</ingredient>
<ingredient quantity="250" unit="g">Hot Chocolate</ingredient>
</recipe>
<recipe name="Cookies" portions="24">
<ingredient quantity="4" unit="oz">Flour</ingredient>
<ingredient quantity="200" unit="g">Chocolate Buttons</ingredient>
<ingredient quantity="600" unit="g">Sugar</ingredient>
</recipe>
<recipe name="Cake" portions="22">
<ingredient quantity="4" unit="oz">Flour</ingredient>
<ingredient quantity="4" unit="oz">Sugar</ingredient>
<ingredient quantity="4" unit="oz">Butter</ingredient>
<ingredient quantity="60" unit="n/a">Eggs</ingredient>
</recipe>
</UserRecipes>
You can see that I have multiple recipes and the ingredients as child tags.
My form currently looks like this:
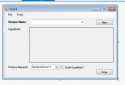
The program consists of a dropdown box for the user to select recipes, a DatGridView control to view/edit the ingredients, an up/down selection box to allow them to enter portion amounts (along with a checkbox that when checked, will scale up the quantities in the ingredients according to the new amount of people selected).
Currently, I have a way to view all the recipes in combobox, and can view either all the ingredients in the XML file in the DataGridView, or all the recipe names/portions in the DataGridView.
I need a way to filter the selected ingredients to only show the ones related to the one selected in the drop down.
My current code is below. Please note, this is no where near finished, but just used so I can get an idea of the techniques I need to use before I create the final program in school.
Code:
Imports System.IO
Imports System.Xml
Public Class Form1
Public xmld As XmlDocument
Public nodelist As XmlNodeList
Public DS As New DataSet
Public DS2 As New DataSet
Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load
xmld = New XmlDocument()
xmld.Load("G:\Computing\!Recipe Test\XMLTest.xml")
nodelist = xmld.SelectNodes("/UserRecipes/recipe")
For Each node In nodelist
Dim recipeName = node.Attributes.GetNamedItem("name").Value
ComboBox1.Items.Add(recipeName)
Next
Dim xmlFile = XmlReader.Create("G:\Computing\!Recipe Test\XMLTest.xml", New XmlReaderSettings())
DS.ReadXml(xmlFile, XmlReadMode.Auto)
Dim dvRecipes As DataView = DS.Tables(0).DefaultView
DataGridView1.DataSource = dvRecipes
xmlFile.Close()
End Sub
Private Sub ComboBox1_SelectedIndexChanged(sender As Object, e As EventArgs) Handles ComboBox1.SelectedIndexChanged
Dim dvRecipes As DataView = DS.Tables(0).DefaultView
dvRecipes.RowFilter = "name='" & ComboBox1.Text & "'"
End Sub
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
DS.WriteXml("G:\Computing\!Recipe Test\XMLTest.xml")
End Sub
End Class
My changing the index in this line from 0 to 1:
Code:
Dim dvRecipes As DataView = DS.Tables(0).DefaultView
I've hit a brick wall with this, and need all the help I can get.
Many, many thanks!
Stephen