This is a very simple program, but one of my first proper programs that actually does something - albeit not that useful... unless you're a spy. I would have to write a security related program ....
CYPHER - Creates a secret "code" using an XOR Cypher. Cypher key is a typed password, which is then used to produce the coded or decoded output.
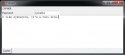
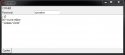
I've updated the program to display *'s in the Password box, after rummaging through the tkinter references.
CYPHER - Creates a secret "code" using an XOR Cypher. Cypher key is a typed password, which is then used to produce the coded or decoded output.
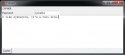
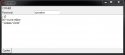
I've updated the program to display *'s in the Password box, after rummaging through the tkinter references.
Code:
# Cypher v1.0
# Python version 3.2
#
# Created by Will Watts
# Secret Code generator using a XOR cypher.
from tkinter import *
from itertools import zip_longest
class Application(Frame):
""" GUI application for XOR Cypher. """
def __init__(self, master):
""" Initialize Frame. """
super(Application, self).__init__(master)
self.grid()
self.create_widgets()
def create_widgets(self):
""" Create widgets to get password and message and display code. """
#Instruction label
Label(self,
text = "CYPHER"
).grid(row = 0, column = 0, sticky = W)
#password label
Label(self,
text = "Password:"
).grid(row = 3, column = 0, sticky = W)
self.password_ent = Entry(self, show="*")
self.password_ent.grid(row = 3, column = 1, sticky = W)
#message box
self.message_txt = Text(self,
width = 75,
height = 10,
wrap = WORD)
self.message_txt.grid(row = 5, column = 0, columnspan = 4)
#cypher button
Button(self,
text = "Cypher",
command = self.cypher
).grid(row = 8, column = 0, sticky = W)
def cypher(self):
key = self.password_ent.get()
data = self.message_txt.get(0.0, END)
coded = ''.join(chr(ord(x) ^ ord(y)) for (x,y) in zip_longest(data, key, fillvalue = '-'))
self.message_txt.delete(0.0, END)
self.message_txt.insert(0.0, coded)
root = Tk()
root.title("CYPHER")
app = Application(root)
root.mainloop()